How to start using Hidden Markov model in Python to automate music transcription?
You will first need to install the necessary libraries and dependencies. The easiest way to do this is by using the pip package manager, which is included with most Python installations. Once you have pip installed, you can use it to install the hmmlearn library, which provides a set of functions and classes for working with hidden Markov models in Python.
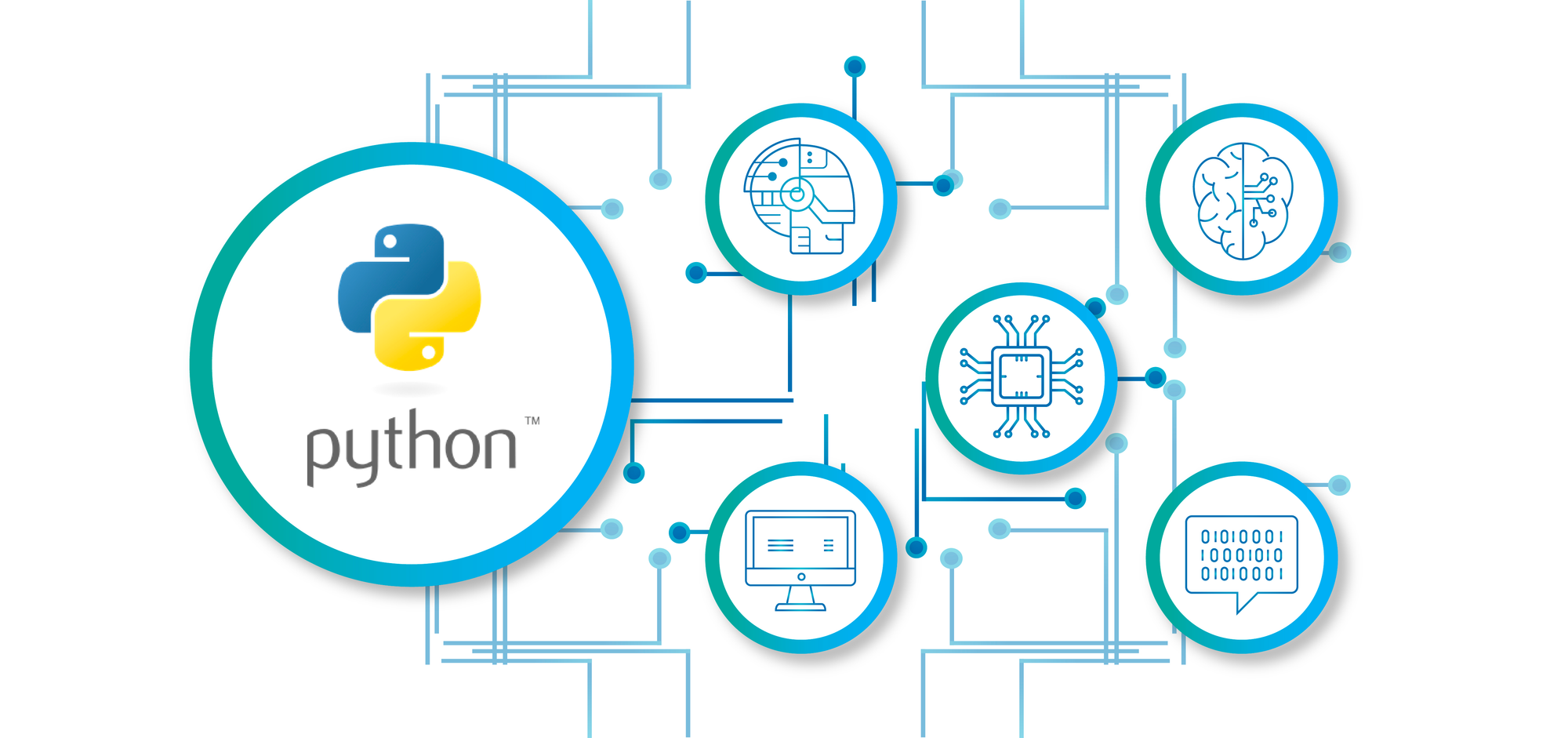
Once you have installed the hmmlearn library, you can use it to create and train a hidden Markov model. To do this, you will need to import the relevant classes and functions from the library, and then use them to define and train your model. For example, the following code creates a simple hidden Markov model using the GaussianHMM class from the hmmlearn library:
from hmmlearn import hmm
# Define the model
model = hmm.GaussianHMM(n_components=2)
# Train the model
model.fit([[1], [2], [3]])
# Use the model to make predictions
predictions = model.predict([[1], [2], [3]])
This code creates a hidden Markov model with two hidden states, trains the model using a series of musical notes, and then uses the trained model to make predictions about the likelihood of certain sequences of notes. Of course, this is just a simple example, and in practice you will need to experiment with different settings and configurations to find the best model for your specific use case.
How to train Markov model to transcribe music?
To train a Markov model to transcribe music, you will need to follow these basic steps:
- Convert the audio data into a series of discrete musical events, such as individual notes or chords. This typically involves using signal processing techniques to analyze the audio data and identify the individual notes or events that make up the music.
- Define the Markov model that you will use for transcription. This typically involves selecting the specific type of Markov model that you want to use (e.g. hidden Markov model, Markov chain, etc.), as well as defining the number of hidden states and other model parameters.
- Train the Markov model using the musical data that you prepared in step 1. This typically involves feeding the data into the model and letting the model "learn" the underlying patterns and relationships in the data.
- Use the trained Markov model to transcribe new music. This typically involves feeding the audio data for the new music into the model, and using the trained model to make predictions about the sequence of musical events in the data.
How to convert the audio data into a series of discrete musical events?
To convert audio data into a series of discrete musical events, you will need to use signal processing techniques to analyze the audio data and identify the individual notes or events that make up the music, some common approaches include:
- Using a pitch detection algorithm to identify the individual notes in the music. This approach involves analyzing the audio data to determine the fundamental frequency of each note, and then mapping these frequencies to specific musical pitches.
- Using a beat tracking algorithm to identify the rhythm and tempo of the music. This approach involves analyzing the audio data to determine the underlying beat structure of the music, and then using this information to segment the audio data into individual musical events.
- Using a machine learning algorithm to learn the patterns and relationships in the audio data. This approach involves training a machine learning model on a large dataset of musical audio data, and then using the trained model to transcribe new music.
To do that in Python, you can use the librosa library, which provides a set of tools for working with audio data in Python. Using librosa, you can easily load audio data from a file and then use various functions and algorithms to analyze the data and identify the individual notes or events that make up the music.
For example, the following code uses the librosa library to load an audio file and then uses the librosa.piptrack
function to identify the pitch of each note in the audio data:
import librosa
# Load the audio data from a file
audio, sr = librosa.load('song.mp3')
# Use the librosa.piptrack function to identify the pitch of each note
pitches, magnitudes = librosa.piptrack(audio, sr)
This code loads the audio data from a file, and then uses the librosa.piptrack
function to identify the pitch of each note in the audio data. The pitches and magnitudes of each note are returned as a NumPy array, which you can then use to transcribe the music.